Over the past few days I’ve been working on a simple TFS utility that will provide the ability to import and/or export Areas and Iterations. The overall intent of this exercise is not only to reinvent the wheel but to also gain some experience in writing Visual Studio Add-ins. At the same time, I am planning on posting “interesting” tidbits of code along the way.
Currently, I am still in the phase of writing the utility (it will first be a standalone utility and later, a Visual Studio Add-in) and had the desire to display the Connect to Team Foundation Server dialog…
I suspected there was an API for this dialog; I only needed to find it – and I did. Paul Hacker has a nice post that explains the basics for achieving this goal using the DomainProjectPicker class. His post explains very nicely how to get it to display the dialog in one mode – that of allowing only a single project to be selected from the dialog. However, there are several variations on what type of functionality is available based upon how you call the API. The following sections cover the various scenarios for displaying the Connect to Team Foundation Server dialog.
Server Selection Only
This mode allows you to select a Team Foundation Server that has been previously registered and/or allows you to register a new Team Foundation Server. By default, the resulting dialog looks like this:
The code to display this dialog is fairly simple:
public void DisplayTfsConnectionDialog()
{
// Initialize the TFS project picker object
DomainProjectPicker projectPicker = new DomainProjectPicker(DomainProjectPickerMode.None);
// Display the dialog
if (projectPicker.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
// Do something...
}
}
Notice the argument value passed into the DomainProjectPicker constructor – DomainProjectPickerMode.None.
You can initialize the selected server by setting the project picker’s SelectedServer property. To set a default server, add the following lines of code to the above example somewhere prior to calling the ShowDialog method:
// Select a default server
TeamFoundationServer defaultServer = TeamFoundationServerFactory.GetServer("tfs-dev");
projectPicker.SelectedServer = defaultServer;
I’ve hard-coded the server name (“tfs-dev”) for example purposes. When the modified code is ran, the following dialog is displayed:
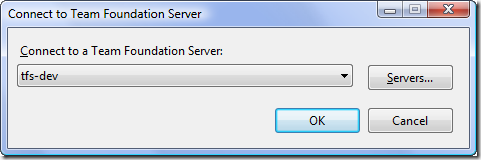
The SelectedServer property returns an instance of TeamFoundationServer for the selected server.
Single Project Selection
This mode allows you to select a team project as well as a server. In this mode, you can select only one project. The resulting dialog looks like this:
You need to make one simple modification to the code example above to display this version of the dialog – change the DomainProjectPicker constructor argument to DomainProjectPickerMode.AllowProjectSelect. To retrieve the name of the selected project, just check the SelectedProjects property. This property returns an array (there will be only one element in this case) of ProjectInfo objects. For example, to display the name of the selected project, modify the above example accordingly:
// Display the dialog
if (projectPicker.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
MessageBox.Show(projectPicker.SelectedProjects[0].Name, "Selected Project");
}
Multiple Project Selection
This mode is similar to the single project mode above except it allows you to select one or more projects. In this case, you must combine two values for the DomainProjectPicker constructor argument: DomainProjectPickerMode.AllowProjectSelect | DomainProjectPickerMode.AllowMultiSelect. This will result in a dialog like this one:
You can retrieve the selected project(s) by accessing the SelectedProjects property just like in the example above with the exception that there may be more than one element in the array.
Disabling Server Selection
You can also combine the DomainProjectPicker constructor arguments as listed above with the value DomainProjectPickerMode.DisableServerSelect. This has the effect of disabling the Server drop-down list and the Servers… button on the dialog. For example:
Selecting a Default Project
Just as you can select a default Team Foundation Server, you can also select one or more default projects. There is one caveat - you can only select default projects if you’re using Multiple Project Selection mode. I really don’t understand why the creators of this particular API chose not to allow a default project selection for Single Selection Mode, but that’s just the way it is.
To set one or more default projects, you must initialize the DefaultSelectedProjects property. This property is a SortedList where the key is the URL of a specific Team Foundation Server and the value is an ArrayList of projects to be selected for the server defined by the key. Here is a code example that selects the “Demo” project by default when displaying the connection dialog:
// Initialize the TFS project picker object
DomainProjectPicker projectPicker = new DomainProjectPicker(DomainProjectPickerMode.AllowProjectSelect | DomainProjectPickerMode.AllowMultiSelect);
// Select a default server
TeamFoundationServer defaultServer = TeamFoundationServerFactory.GetServer("tfs-dev");
projectPicker.SelectedServer = defaultServer;
// Select a default project (in this case, the "Demo" project)
ICommonStructureService css = (ICommonStructureService)defaultServer.GetService(typeof(ICommonStructureService));
SortedList defaultServerList = new SortedList();
ArrayList defaultProjectList = new ArrayList();
defaultProjectList.Add(css.GetProjectFromName("Demo").Uri.ToString());
defaultServerList.Add(defaultServer.Uri.ToString(), defaultProjectList);
projectPicker.DefaultSelectedProjects = defaultServerList;
// Display the dialog
if (projectPicker.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
MessageBox.Show(projectPicker.SelectedProjects[0].Name, "Selected Project");
}
The following dialog is displayed using the above code example:
That pretty much covers the various scenarios. Hopefully the above code examples will help out the next person looking for similar functionality.